Kotlin API Client
This library allows you to request recommendations and send interactions between users and items (such as views, bookmarks, or purchases) to Recombee. It is a thin wrapper around the Recombee API and provides a simple way to interact with it.
This SDK is designed for usage in Android applications or other client-side applications (e.g. Compose Multiplatform).
For server-side integration with the Recombee API, use our dedicated Java library or other available server-side SDKs.
For security reasons, it is not possible to change the item catalog, such as the properties of items, using this SDK. To send your Catalog to Recombee, use one of the following methods:
- Use one of our server-side SDKs, for example using a script which runs periodically (see Managing Item Catalog for more details),
- Or set up a Catalog Feed in the Admin UI.
Install
The client is available in the Maven Central Repository, which is included in new Android projects by default.
Adding the client into your project is therefore as simple as adding the dependency into your build.gradle
:
dependencies {
implementation("com.recombee:apiclientkotlin:5.0.0")
}
--- libs.versions.toml ---
[versions]
recombee = "5.0.0"
[libraries]
recombee = { group = "com.recombee", name = "apiclientkotlin", version.ref = "recombee" }
--- build.gradle.kts ---
dependencies {
implementation(libs.recombee)
}
dependencies {
implementation "com.recombee:apiclientkotlin:5.0.0"
}
You can then find all the classes and methods in the com.recombee.apiclientkotlin
package and its subpackages.
Configure
In order to use the API, you will need to create an instance of the RecombeeClient
class. You will need:
- the ID of your database,
- the public token.
You can find these in the Admin UI, in your Database's Settings page, under API ID & Tokens. Along with this information, you can also find the full code snippet for initializing the client, including the above-mentioned parameters.
Ideally, you should only have one instance of the RecombeeClient
in your application, as it is a lightweight object and can be reused for multiple requests.
We published a simple Android example app to help you with the integration. Feel free to use it as a reference.
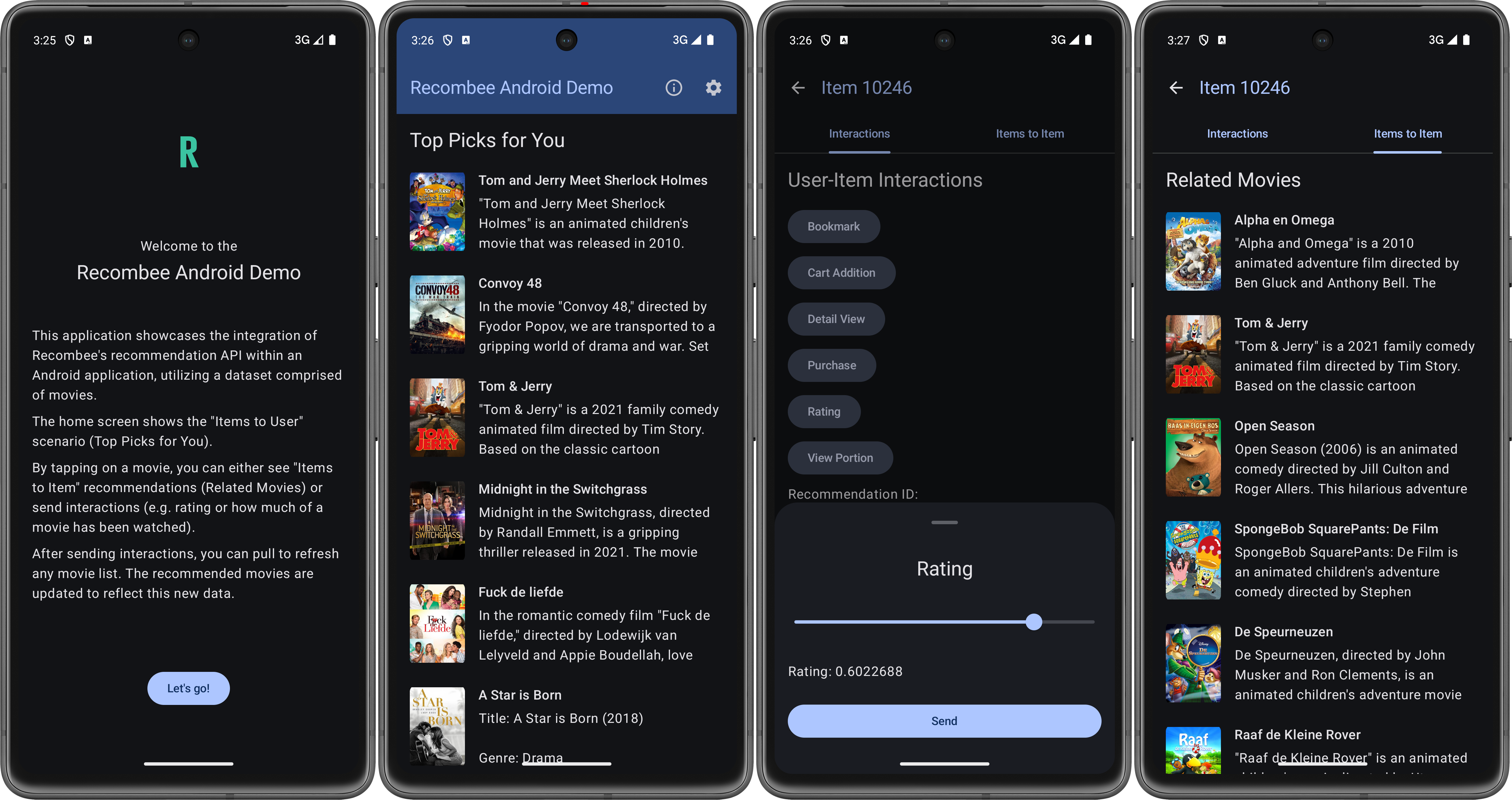
You can initialize the client as follows:
import com.recombee.apiclientkotlin.RecombeeClient
import com.recombee.apiclientkotlin.util.Region
// Initialize the API client with the ID of your database and the associated PUBLIC token
val client = RecombeeClient(
databaseId = "database-id",
publicToken = "...db-public-token...",
region = Region.UsWest // the region of your database
)
You can also set several optional parameters when initializing the client:
val client = RecombeeClient(
// Required parameters:
databaseId = "database-id",
publicToken = "...db-public-token...",
// Optional parameters:
// Use this if you were assigned a custom URI by the Recombee Support team (default: null)
baseUri = "custom-uri.recombee.com",
// The port to connect to (default: null)
port = 443,
// Whether to use HTTPS - can be used for debugging (default: true)
useHttpsByDefault = true,
)
Send Interactions
After you have initialized the client, you can send interactions between users and items.
The individual interactions are classes within the com.recombee.apiclientkotlin.requests
package.
After you create an instance of the interaction, you can send it using one of two methods of the RecombeeClient
class:
send
- for callbackssendAsync
- for coroutines (must be called from inside aCoroutineScope
)
Each interaction has both mandatory and optional parameters.
The most important optional parameter is recommId
- the ID of the recommendation to which the interaction belongs.
Providing this ID allows you to track successful recommendations.
For more information, read about Reported Metrics.
For a full list of interactions, along with their parameters, refer to the API Reference.
import com.recombee.apiclientkotlin.requests.*
// Either create the interaction first and then send it
val bookmark = AddBookmark(userId = "user-13434", itemId = "item-256")
client.send(bookmark)
// Or send it directly
client.send(
AddCartAddition(
userId = "user-4395",
itemId = "item-129",
recommId = "23eaa09b-0e24-4487-ba9c-8e255feb01bb",
)
)
client.send(AddDetailView(userId = "user-9318", itemId = "item-108"))
client.send(AddPurchase(userId = "user-7499", itemId = "item-750"))
client.send(AddRating(userId = "user-3967", itemId = "item-365", rating = 0.5))
client.send(SetViewPortion(userId = "user-4289", itemId = "item-487", portion = 0.3))
If you want to send multiple interactions at once, you can use the Batch
request:
import com.recombee.apiclientkotlin.requests.*
val batch = Batch(listOf(
AddBookmark(userId = "user-13434", itemId = "item-256"),
AddCartAddition(userId = "user-4395", itemId = "item-129", cascadeCreate = true),
AddDetailView(userId = "user-9318", itemId = "item-108"),
))
client.send(batch)
You can then use callbacks (or Result
) to handle any exceptions that may occur.
client.send(
AddRating(
userId = "user-3967",
itemId = "item-365",
rating = 0.5,
cascadeCreate = true
),
{ println("Interaction sent successfully") },
{ exception: ApiException ->
println("Exception: $exception")
// use fallback ...
}
)
val response = client.sendAsync(
AddRating(
userId = "user-3967",
itemId = "item-365",
rating = 0.5,
cascadeCreate = true
)
)
if (response.isFailure) {
println("Exception: ${response.exceptionOrNull()}")
// use fallback ...
} else {
println("Interaction sent successfully")
}
Get Recommendations
With an initialized client, you can also request recommendations.
There are multiple types of recommendations, such as:
- Recommend Items to User,
- Recommend Items to Item,
- Recommend Item Segments to User (these can be categories, genres, artists, etc.),
- or others.
Each recommendation request is a class within the com.recombee.apiclientkotlin.requests
package.
After you create an instance of the recommendation request, you can send it using the send
or sendAsync
methods of the client (depending on whether you want to use callbacks or coroutines).
val request = RecommendItemsToUser(
userId = "user-x",
count = 10,
scenario = "homepage-for-you"
)
client.send(request,
{ recommendationResponse: RecommendationResponse ->
println("recommId: ${recommendationResponse.recommId}")
for (recommendedItem in recommendationResponse.recomms) {
println("ID: ${recommendedItem.id}")
}
},
{ exception: ApiException ->
println("Exception: $exception")
// use fallback ...
}
)
val request = RecommendItemsToUser(
userId = "user-x",
count = 10,
scenario = "homepage-for-you"
)
val response = client.sendAsync(request)
response.onSuccess { recommendationResponse: RecommendationResponse ->
println("recommId: ${recommendationResponse.recommId}")
for (recommendedItem in recommendationResponse.recomms) {
println("ID: ${recommendedItem.id}")
}
}.onFailure { exception -> // ApiException
println("Exception: $exception")
// use fallback ...
}
For a full list of request parameters and possible responses, visit the API Reference.
Personalized Search
Personalized full-text search is requested in the same way as recommendations:
val searchQuery = " ... search query from search field ...."
val request = SearchItems(
userId = "user-x",
searchQuery = searchQuery,
count = 10,
scenario = "search",
returnProperties = true
)
client.send(request,
{ searchResponse: SearchResponse ->
println("recommId: ${searchResponse.recommId}")
for (recommendedItem in searchResponse.recomms) {
println("ID: ${recommendedItem.id} Values: ${recommendedItem.getValues()}")
}
},
{ exception: ApiException ->
println("Exception: $exception")
// use fallback ...
}
)
val searchQuery = " ... search query from search field ...."
val request = SearchItems(
userId = "user-x",
searchQuery = searchQuery,
count = 10,
scenario = "search",
returnProperties = true
)
val response = client.sendAsync(request)
response.onSuccess { searchResponse: SearchResponse ->
println("recommId: ${searchResponse.recommId}")
for (recommendedItem in searchResponse.recomms) {
println("ID: ${recommendedItem.id} Values: ${recommendedItem.getValues()}")
}
}.onFailure { exception -> // ApiException
println("Exception: $exception")
// use fallback ...
}
Recommend Next Items
If you are implementing features like infinite scroll or pagination, you can use the RecommendNextItems
request to load recommendations progressively.
This means you can fetch the next set of recommended items without repeating the ones you have already displayed.
To use this functionality, you must provide the recommId
from the initial recommendation request.
For more details, see the Recommend Next Items documentation.
var initialRecommId: String? = null
// Fetch the initial set of 5 recommendations for user-13434
val initialRecomms = client.sendAsync(RecommendItemsToUser("user-1", 5))
initialRecomms.onSuccess { it ->
// Store the recommId to be used by the next request
initialRecommId = it.recommId
// Display recommendations
}
// Get the next 3 recommendations as user-13434 scrolls down
initialRecommId?.let { recommId ->
val nextRecomms = client.sendAsync(RecommendNextItems(recommId, 5))
nextRecomms.onSuccess { TODO() }
}
Batch Requests
You may encounter a situation where you display recommendations in multiple places on your website.
In such cases, you can use the Batch
request to send multiple recommendation requests at once. This can help reduce the number of HTTP requests and improve performance.
For example, you can request the most popular items, as well as items related to a specific user or item, in a single Batch:
val batch =
Batch(
requests =
listOf(
RecommendItemsToItem(
targetUserId = "user-13434",
itemId = "item-356",
count = 10,
scenario = "because-you-watched",
returnProperties = true,
includedProperties = listOf("title", "images"),
),
RecommendItemsToUser(
userId = "user-13434",
count = 10,
scenario = "new-releases",
returnProperties = true,
includedProperties = listOf("title", "images"),
),
RecommendItemsToUser(
userId = "user-13434",
count = 10,
scenario = "popular",
returnProperties = true,
includedProperties = listOf("title", "images"),
),
),
distinctRecomms = true,
)
val result = client.sendAsync(batch)
if (result.isFailure) {
return listOf()
}
val data = result.getOrElse { listOf() }
val sections: List<List<Item>> =
data.map {
if (!it.successful) listOf()
else {
val response = it.getResponse() as RecommendationResponse
response.recomms.map { item ->
Item(
id = item.id,
title = item.getValues()["title"] as? String ?: "",
images = item.getValues()["images"] as? List<String> ?: listOf(),
recommId = response.recommId,
)
}
}
}
println("Because You Watched: ${sections[0]}")
// Because You Watched: [Item(id=..., title=..., ...), ...]
println("New Releases: ${sections[1]}")
// New Releases: [Item(id=..., title=..., ...), ...]
println("Popular: ${sections[2]}")
// Popular: [Item(id=..., title=..., ...), ...]
The optional parameter distinctRecomms
of the Batch
ensures that the recommended items are not repeated across the responses.
You can find more information about Batch requests in the API Reference.
Optional Parameters
Recommendation requests support various optional parameters to customize their behavior. For a comprehensive list, refer to the API Reference. Below is an example showcasing some commonly used parameters:
val request = RecommendItemsToUser(
userId = "user-13434",
count = 5,
// Scenarios help identify the context where recommendations are displayed
// and can be customized in the Admin UI at https://admin.recombee.com
scenario = "homepage",
// Include detailed properties of the recommended items in the response
returnProperties = true,
// Specify which properties to include (requires returnProperties = true)
includedProperties = listOf("title", "img_url", "url", "price"),
// Apply a ReQL filter to refine recommendations,
// e.g., "Recommend only items with a title that are in stock."
filter = "'title' != null AND 'availability' == \"in stock\""
// Note: You can define scenario-specific filters in the Admin UI.
)
Error Handling
The API client throws exceptions when an error occurs. The exceptions are part of the com.recombee.apiclientkotlin.exceptions
package.
The possible exceptions are:
Exception | Cause |
---|---|
ApiException | Base class for all exceptions |
ResponseException : ApiException | The API returned an error code (e.g. invalid parameter) |
ApiIOException : ApiException | Request failed (e.g. network issues) |
ApiTimeoutException : ApiIOException | Request timed out |
We are doing our best to provide a reliable service, but sometimes things can go wrong. For this reason, we recommend that you always handle exceptions and provide fallbacks in your application.
For example, when requesting recommendations, a fallback could be to display a generic set of items or an error message to the user.